Algorithms - Dynamic Programming
date
Dec 9, 2024
type
Post
AI summary
slug
algorithm-dp
status
Published
tags
Algorithm
summary
Introduction
Dynamic programming (DP) is a powerful technique in computer science and mathematics used to solve problems by breaking them into smaller, simpler subproblems. These subproblems are solved individually, and their solutions are stored to avoid redundant calculations. This method is particularly effective for problems exhibiting two key properties:
- Overlapping Subproblems: The problem can be divided into subproblems that are solved multiple times.
- Optimal Substructure: The solution to a larger problem can be constructed using solutions to its subproblems.
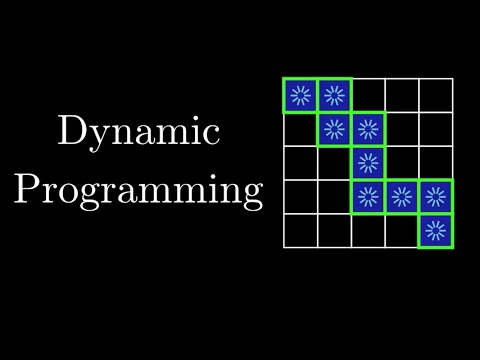
Steps in Dynamic Programming
- Define the Problem: Clearly define the state and how it represents a subproblem.
- Identify the Recursive Relation: Determine how the solution to the current state depends on previous states.
- Base Cases: Specify the simplest cases with known solutions.
- Memoization or Tabulation: Decide whether to use a top-down or bottom-up approach to compute and store results.
- Solve Iteratively or Recursively: Compute the solution to the original problem using the stored subproblem results.
Two Main Approaches
Top-Down (Memoization)
- Description: Solve the problem recursively, caching the results of subproblems in a data structure (e.g., a hash table or array) to avoid recalculating them.
- Steps:
- Start from the original problem.
- Break it into smaller subproblems recursively.
- Store the result of each subproblem as it is computed.
- Reuse stored results for overlapping subproblems.
- Example: Calculating Fibonacci numbers using recursion with memoization.
Bottom-Up (Tabulation)
- Description: Solve the problem iteratively by starting with the smallest subproblems and building up to the original problem. Store results in a table and use them to solve larger subproblems.
- Steps:
- Identify the order of subproblem computation (e.g., smallest to largest).
- Initialize a table with base case solutions.
- Iterate through the table, solving subproblems in order.
- Use previously computed values to fill in the table.
- Example: Computing Fibonacci numbers using an iterative loop.
Applications
Dynamic programming is widely used in areas such as:
- Optimization Problems: E.g., Knapsack problem, coin change problem.
- Graph Algorithms: E.g., shortest path algorithms like Floyd-Warshall.
- String Manipulation: E.g., longest common subsequence (LCS), edit distance.
- Sequence Analysis: E.g., Fibonacci numbers, Catalan numbers.
By leveraging overlapping subproblems and optimal substructure, dynamic programming reduces computational complexity and provides efficient solutions to problems that would otherwise be computationally expensive.